What happens when a website crams your SWF into an area where it doesn’t fit? In this Quick Tip, you’ll find out how to take control of how your SWF scales.
Step 1: Set up Your SWF
Start a new Flash project, and set your stage to be 350px wide, 250px high.
Create a basic document class (see this Quick Tip for more info):
package { import flash.display.Sprite; public class Main extends Sprite { public function Main() { } } }
The FLA (and the SWC, for those of you not using Flash Pro) in the zip file contains two assets:
CheckedBackground, which is the same size as the stage, with its registration point in the top-left corner.
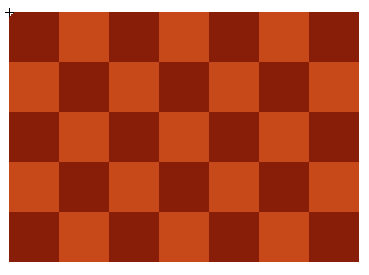
Face, which is about half as wide as the stage, with its registration point in the center.
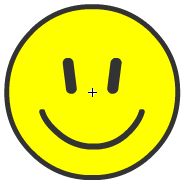
Position them on the stage like so:
public class Main extends Sprite { private var checkedBackground:CheckedBackground; private var face:Face; public function Main() { checkedBackground = new CheckedBackground(); checkedBackground.x = 0; checkedBackground.y = 0; this.addChild( checkedBackground ); face = new Face(); face.x = stage.stageWidth / 2; //center the face horizontally face.y = stage.stageHeight / 2; //center the face vertically this.addChild( face ); } }
Run your SWF:
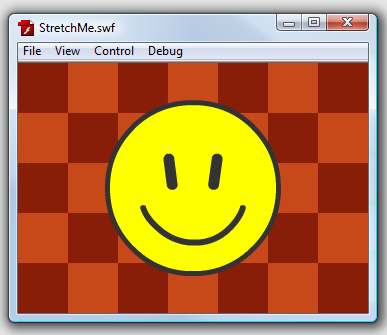
Step 2: Stretch the Player Window
Make the window bigger, make it smaller, stretch it both in and out of proportion, and see how the contents change:
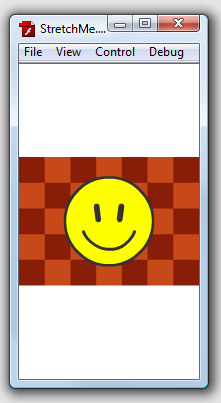
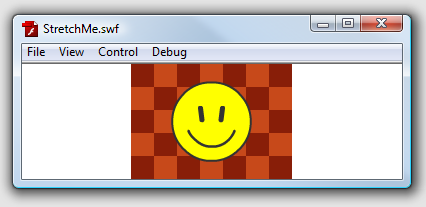
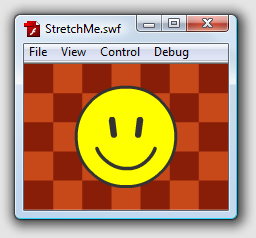
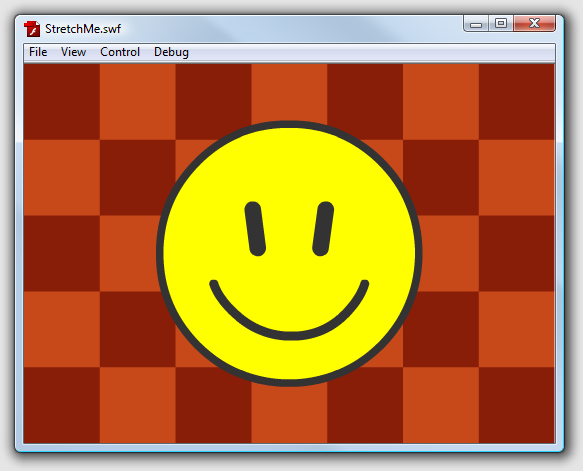
This is Flash’s default scale mode, SHOW_ALL. The contents are never distorted, and you can always see the whole stage. This means you get a “letterbox” effect if stretching out of proportion.
Step 3: Try the NO_BORDER Scale Mode
SHOW_ALL is the default scale mode, but there are three others we can use.
Import the StageScaleMode class:
import flash.display.StageScaleMode;
This contains static consts that can be used to set the scale mode. Let’s try NO_BORDER; add this line to your constructor function:
stage.scaleMode = StageScaleMode.NO_BORDER;
Run your SWF and stretch it again:
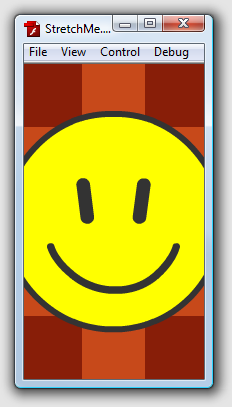
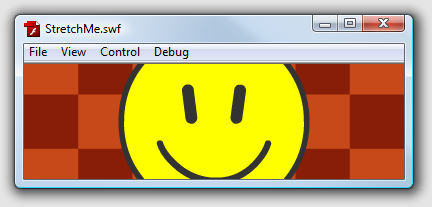
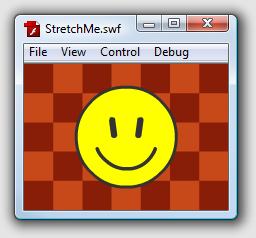
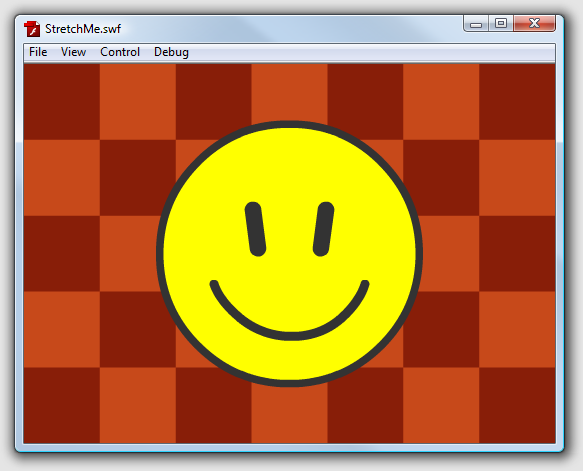
As the name suggests, NO_BORDER avoids the letterbox effect. The contents stay in proportion but always fill the available area, even if that means cropping the edges.
Step 4: Try the EXACT_FIT Scale Mode
Change the line that sets the scale mode like so:
stage.scaleMode = StageScaleMode.EXACT_FIT;
Try it out:
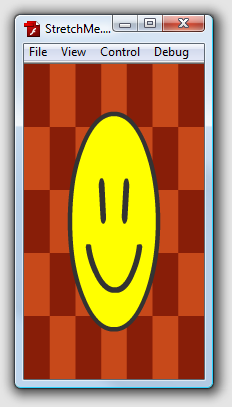
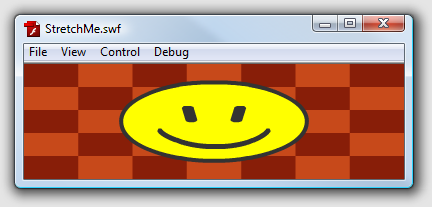
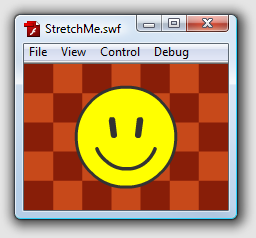
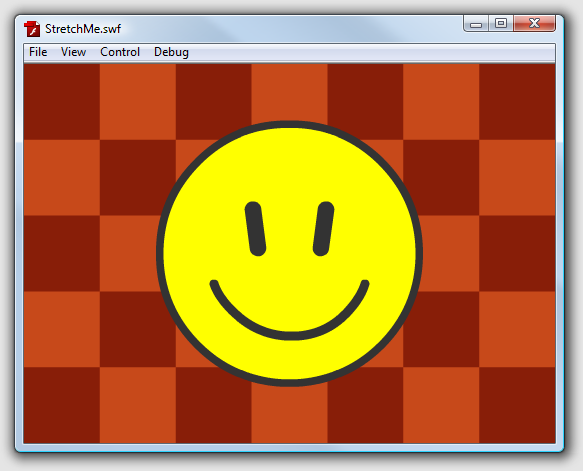
EXACT_FIT makes the edges of the stage stick to the edges of the available area, leading to distortion if the player is stretched out of proportion.
Step 5: Try the NO_SCALE Scale Mode
To check out the final scale mode, change the line like so:
stage.scaleMode = StageScaleMode.NO_SCALE;
Check it out:
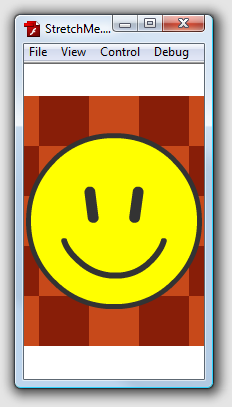
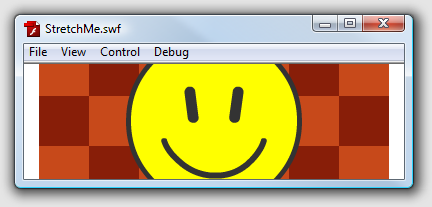
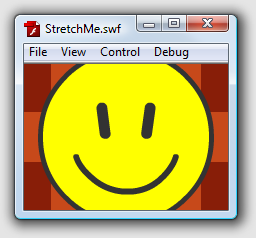
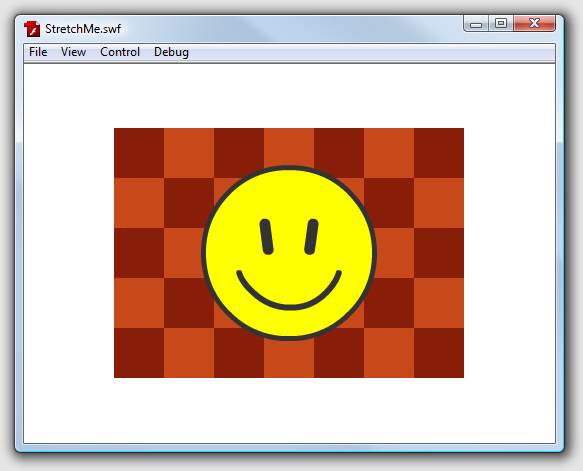
With NO_SCALE, the contents don’t change size at all; they stay centered in the player window, even if that means cropping huge amounts of the edges, or leaving massive borders on all sides.
Conclusion
Stage.scaleMode lets you control how your SWF will appear if a website changes the size of the available area. It’s also useful for creating AIR apps and full screen Web sites; NO_SCALE is a particularly good choice there, as (when combined with a RESIZE event listener) it allows you to fit the entire content to the window, while maintaining the size and proportions of the individual assets.
For more on that, check out Franci Zidar’s series on full screen scalable Web sites