In this Quick Tip, I will show you how to create a basic search application featuring the Google search engine.
Final Result Preview
Let’s take a look at the final result we will be working towards:
Step 1: Brief Overview
Using a TextField and some Events, we’ll send the search terms to Google and display the result in the browser.
Step 2: Set Up Your Flash File
Launch Flash and create a new Flash Document, set the stage size to 320x100px and the background color to #181818.
Step 3: Interface
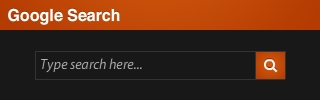
This is the interface we’ll be using, it includes an Input TextField and a button. Set the TextField instance name to searchTerms and the button to searchButton.
Step 4: ActionScript
This is the class that carries out all the work, please read the comments in the code to fully understand its behavior.
package { import flash.display.Sprite; import flash.events.MouseEvent; import flash.events.KeyboardEvent; import flash.ui.Keyboard; import flash.net.navigateToURL; import flash.net.URLRequest; public class Main extends Sprite { public function Main():void { searchButton.addEventListener(MouseEvent.MOUSE_UP, google);//Listens to a button release and executes the search function addEventListener(KeyboardEvent.KEY_DOWN, google);//Listens for the Enter key and executes the search function searchTerms.addEventListener(MouseEvent.MOUSE_DOWN, selectText); //Selects the current text of the textfield for an easy input } //Notice the * (special type), this allow the function to be run by different types of events, avoiding the creation of separate functions with the same code private function google(e:*):void { if(e.type == "mouseUp")//If called by a MouseUp event { navigateToURL(new URLRequest("http://www.google.com/search?q=" + searchTerms.text)); //Search google } else if(e.keyCode == Keyboard.ENTER)//Called by Enter key { navigateToURL(new URLRequest("http://www.google.com/search?q=" + searchTerms.text)); } } private function selectText(e:MouseEvent):void { searchTerms.setSelection(0, searchTerms.length); //Selects the current text in the Textfield } } }
As you can tell by reading the code, the key is the Google URL that lets us add the search terms to the query, this is http://www.google.com/search?q=[search terms here]
.
You can also try this example with different search engines such as Yahoo! for example: http://search.yahoo.com/search;_ylt=?p=[search terms here]
or ActiveTuts: http://active.tutsplus.com/?s=[search terms here].
Step 5: Document Class
Remember to add the class name to the Class field in the Publish section of the Properties panel.
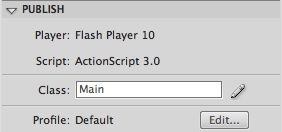
Conclusion
You can create simple and effective search boxes using this technique, try different search engines and create your own search box!
I hope you liked this tutorial, thank you for reading!