In this Quick Tip, I’ll show you how to store and access SharedObjects (the Flash equivalent of cookies) so that you can save and load user data between sessions.
Final Result Preview
Let’s take a look at the final result we will be working towards:
Note that the intro animation doesn’t play if you’ve already seen it once. Try logging in (you can use any username/password combination) and then refresh the browser without logging out. Your details will automatically be entered.
To reset the data saved in the SharedObject, log in and then log out again.
Step 1: Setting up the Document
The first thing to do is download the source files for this tutorial. Since this tutorial is a quick tip, I will skip the layout steps. Once you have the files downloaded, open the ’sharedObject.fla’ file. When the file is loaded, you will notice that we have three keyframes on the timeline.
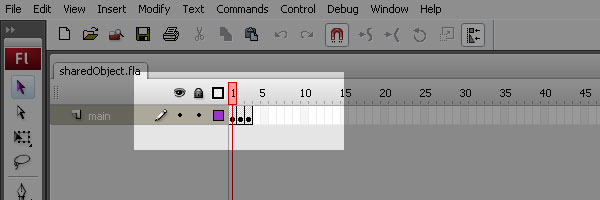
The first keyframe holds the intro animation. The animation is just a movieclip with some motion tweens and a ’stop’ action at the end.
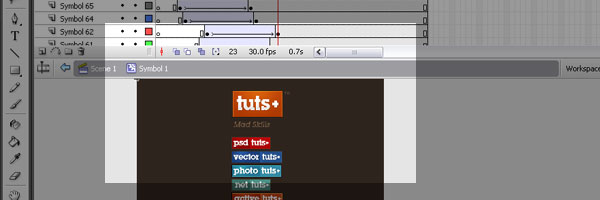
The second keyframe is the login screen. Here a user can enter in their information. They can choose to save it or not. If the user clicks the ‘Watch animation again’ button, they will be taken back to the first keyframe. Also, the SharedObject that we are going to set will be deleted.
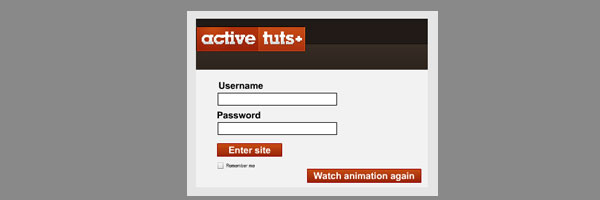
The last keyframe contains a simple RSS reader of the ActiveTuts+ feed. If the user clicks the ‘Log out’ button, they will be taken back to the second keyframe and the SharedObject will be cleared. This means that the user will view the intro animation the next time they visit the site.
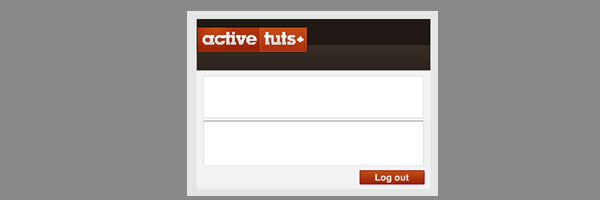
Step 2: The Document Class
Create a new ‘ActionScript’ file and save it to the same folder as ’sharedObject.fla.’ Give the file a name of ’sharedObject.as.’ Next, link the Flash file and ActionScript file together in the Properties panel. For a more in-depth look at how to set up the Document class, visit this other Quick Tip.
Step 3: The Imports
Here are the import statements that we will be using for this file. Since we are using more than one frame of the timeline, we will need to extend it as a MovieClip.
package { import flash.display.MovieClip; import flash.display.SimpleButton; import flash.events.Event; import flash.events.MouseEvent; import flash.net.SharedObject; import flash.net.URLLoader; import flash.text.TextField; public class sharedObject extends MovieClip { public function sharedObject() { } } }
Step 4: Starting With the SharedObject
Here is what the ActionScript 3.0 Language Reference says about SharedObjects:
The SharedObject class is used to read and store limited amounts of data on a user’s computer or on a server. Shared objects offer real-time data sharing between multiple client SWF files and objects that are persistent on the local computer or remote server. Local shared objects are similar to browser cookies and remote shared objects are similar to real-time data transfer devices. To use remote shared objects, you need Adobe Flash Media Server.
In this example, we will only be working with local shared objects. In order to get to started with SharedObjects, we create a variable called ’shared’ and cast it as a SharedObject. Next, we use the ‘getLocal’ method of the SharedObject class. I gave it a name of ‘example,’ but you can give it any name you like.
After we have initialized our SharedObject, we call the ‘init’ function. In the ‘init’ function, we stop the main timeline. We also check the SharedObject to see if the intro animation has been watched. If it has, we send the user to frame 2. If ‘watched’ property on the ‘data’ object of our SharedObject hasn’t been set, we play the animation and listen for it to finish using an ENTER_FRAME event.
package { import flash.display.MovieClip; import flash.display.SimpleButton; import flash.events.Event; import flash.events.MouseEvent; import flash.net.SharedObject; import flash.net.URLLoader; import flash.text.TextField; public class sharedObject extends MovieClip { private var shared:SharedObject; public function sharedObject() { shared = SharedObject.getLocal("example"); init(); } private function init():void { this.stop(); if(shared.data.watched === true) { this.gotoAndStop(2); frame2handler(); } else { this.addEventListener(Event.ENTER_FRAME, onEnter); } } } }
Step 5: Handling the Intro Animation
In the ‘onEnter’ function, we listen to see if the animation has reached the end of its frames. Once it has, we remove the event listener, go to the second keyframe on the main timeline, and call the ‘frame2handler’ function. We also set the ‘watched’ property on the ‘data’ object of the SharedObject. Since ‘data’ is an object, we can assign any value to it. I just used ‘watched’ as an indicator for the intro animation.
Next, we call the ‘flush’ method of the SharedObject. This will save the SharedObject to the appropriate local file and be accessible for later use.
private function onEnter(event:Event):void { if(animation.currentFrame === animation.totalFrames) { this.removeEventListener(Event.ENTER_FRAME, onEnter); this.gotoAndStop(2); frame2handler(); shared.data.watched = true; shared.flush(); } }
Step 6: The Login Screen
In the ‘frame2handler’ function, you’ll notice that I’m calling the ‘addFrameScript’ method. Using this method, we can access different MovieClips in different parts of the timeline. ‘addFrameScript’ is zero based, so in order to access the MovieClips on frame 2, we pass it 1. Also, we pass it an inline function to handle any logic on frame 2. Inside that function, we are checking to see if the SharedObject has the ‘user,’ ‘password’ and ‘remember’ values set. If they are, we populate the text fields with the appropriate information.
private function frame2handler():void { this.addFrameScript(1, function(){ if(shared.data.user != null && shared.data.password != null) { user.text = shared.data.user; password.text = shared.data.password; remember.selected = shared.data.remember; } remember.label = "Remember me"; enter.addEventListener(MouseEvent.CLICK, onClick); watcher.addEventListener(MouseEvent.CLICK, onClick); }); }
Step 7: Handling the Button Clicks
Since the app is small, we’re going to handle all the clicks with one function. Inside the ‘onClick’ function, we check the name of the target of the event. If the name is ‘enter,’ we then check to see if the user wanted their login info remembered. If they did, we simply add more values to the ‘data’ object. If not, we will delete those values from the ‘data’ object. After that, we send the user onto frame 3 and call the ‘frame3handler.’ If the user has clicked the ‘watcher’ button, we delete the value associated with the intro animation. The user then returns to the first frame, and we call the ‘init’ function using ‘addFrameScript.’ Finally, on the third frame, if the user clicks the ‘clearer’ button, we clear the ‘data’ object and all the values of the SharedObject are erased. The user is then sent back to frame 2 and none of their information is retained.
private function onClick(event:MouseEvent):void { switch(event.target.name) { case "enter" : if(remember.selected) { shared.data.user = user.text; shared.data.password = password.text; shared.data.remember = remember.selected; shared.flush(); } else { delete shared.data.user; delete shared.data.password; } this.gotoAndStop(3); frame3handler(); break; case "watcher" : delete shared.data.watched; this.gotoAndStop(1); this.addFrameScript(0, function(){ init(); }); break; case "clearer" : shared.clear(); this.gotoAndStop(2); break; } }
Step 8: Frame 3
In the ‘frame3handler’ function, we use ‘addFrameScript’ again to access the MovieClips on frame 3. Inside of the inline function, we load the RSS feed and use the List and TextArea components to display the information.
private function frame3handler():void { this.addFrameScript(2, function(){ clearer.addEventListener(MouseEvent.CLICK, onClick); var url:URLLoader = new URLLoader(); url.addEventListener(Event.COMPLETE, function(){ var xml:XML = new XML(url.data); var xmlList:XMLList = xml..item; for(var i:int = 0; i<xmlList.length(); i++) { list.addItem({label:xmlList[i].title.text(), data:xmlList[i].description.text()}); } list.selectedIndex = 0; area.htmlText = list.selectedItem.data; list.addEventListener(Event.CHANGE, function(){ area.htmlText = list.selectedItem.data; }); }); url.load(new URLRequest("http://feeds.feedburner.com/flashtuts")); }); }
Conclusion
There is a ton of applications for SharedObjects. Just check out any site like Pandora or game sites like on Adult Swim. However, the best way to learn is to experiment yourself and, of course, subscribe to Tuts+.
Thanks for following along!