If you’re unaware, Envato has a stable and fantastic API to work with that is super powerful and super simple. The latest version (at the time of writing) is release v2. In this article, we’ll review how to access every single public set from the Envato API.
Learning the Basics
First things first: you need to know where to find the API, what it is, and how exactly it works. You can find the official API release on the forums, as well as the update to the API thread and the v1 update information.
The API works by making requests to custom URLs containing data that you wish to be returned. The data can also be returned in two formats, xml or JSON, which is returned is up to you. I prefer returning as JSON and using PHPs json_decode to turn the data into nested arrays.
What we will Accomplish
Below, you will see a screenshot of all of the currently available public sets. We will go through each and everyone of these today, individually, and with a working code example and preview of the final result!
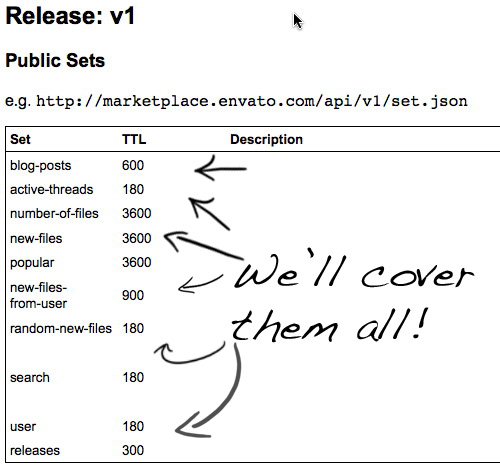
For each set we cover today, I will briefly describe what the point of the set is, post the code, and then later explain each step of the code below. Be sure to ask any questions about any of the code snippets you find below.
Also, feel free to use the this code and techniques learned in this tutorial however you wish!
1. Blog Posts
The blog-posts set allows you to query and display a list of blog posts for any particular market. It requires one parameter, which is the marketplace you would like the recent blog posts from.
//Initialize curl $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://marketplace.envato.com/api/v1/blog-posts:themeforest.json'); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $ch_data = curl_exec($ch); curl_close($ch); if(!empty($ch_data)) { $json_data = json_decode($ch_data, true); //print_r($json_data); $data_count = count($json_data['blog-posts']) -1; echo '
- ';
for($i = 0; $i <= $data_count; $i++)
{
echo '
- ',$json_data['blog-posts'][$i]['title'],' '; } echo '
Lets walk through the above snippet in detail, as the rest of our snippets will look very similar.
- We start off by initiating a new curl handle. cURL will allows us to query the API with whatever parameters we like. You could use file_get_contents, but you will gain a performance boost with cURL.
- We set our target URL. Notice how we passed in the set blog-posts and then themeforest for the parameter. Also, note we are requesting the data is JSON format.
- Next, we set two more cURL options. CURLOPT_CONNECTTIMEOUT allows us to set the timeout time for our request, which we set to 5 seconds. The other option is CURLOPT_RETURNTRANSFER tells cURL to return the data as a string instead of outputting it directly.
- We store the results of the cURL request in $ch_data.
- Now we check if there was indeed some data returned, if so we decode the json and turn it into a nested array.
- Lastly, we loop through the array elements and print out some basic data.
Keep these details in mind; like I said, you will see this pattern in almost every set we cover, though they will vary slightly.
And a demo of the output:
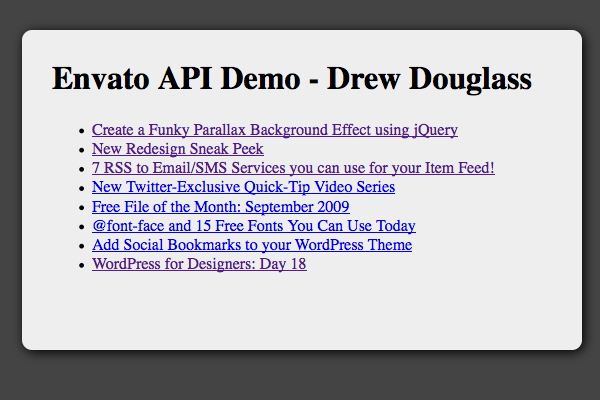
2. Active Threads
The active-threads set allows you to pull out some recently active forum threads.
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://marketplace.envato.com/api/v1/active-threads:themeforest.json'); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $ch_data = curl_exec($ch); curl_close($ch); if(!empty($ch_data)) { $json_data = json_decode($ch_data, true); //print_r($json_data); $data_count = count($json_data['active-threads']) -1; echo '
- ';
for($i = 0; $i <= $data_count; $i++)
{
echo '
- ',$json_data['active-threads'][$i]['title'],' '; } echo '
Note a few things here; one, we have changed our request URL to reflect the new set we want to obtain. Secondly, note the array namings have changed as they will with each new set we request. Lastly, notice how print_r is commented out. This is very helpful for debugging and viewing the structure and hierarchy of the data.
And a demo of the output:
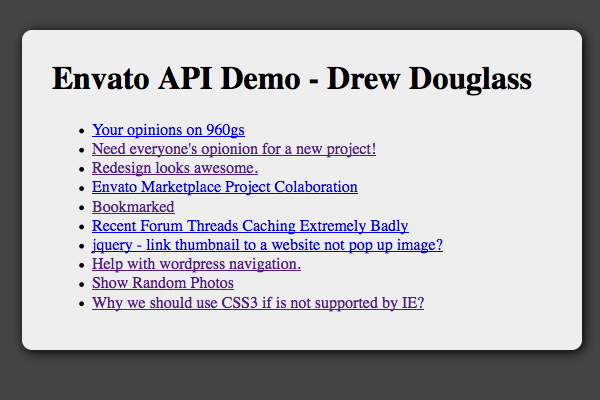
3. Number of Files
Don’t let the name of the number-of-files set fool you. It is not the number of a users files (but we will cover this!) but rather the number of files in a given category from a given market. For example, this would let you find out how many total site templates we have on ThemeForest, which we’ll do now.
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://marketplace.envato.com/api/v1/number-of-files:themeforest.json'); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $ch_data = curl_exec($ch); curl_close($ch); if(!empty($ch_data)) { $json_data = json_decode($ch_data, true); //print_r($json_data); $data_count = count($json_data['number-of-files']) -1; echo '
- ';
for($i = 0; $i <= $data_count; $i++)
{
echo '
- ',$json_data['number-of-files'][$i]['category'],' - ',$json_data['number-of-files'][$i]['number_of_files'],' '; } echo '
Of course, our URL request has changed and we have passed the parameter of themeforest, which is the marketplace we will pull the data from. An example of the output from this snippet is below:
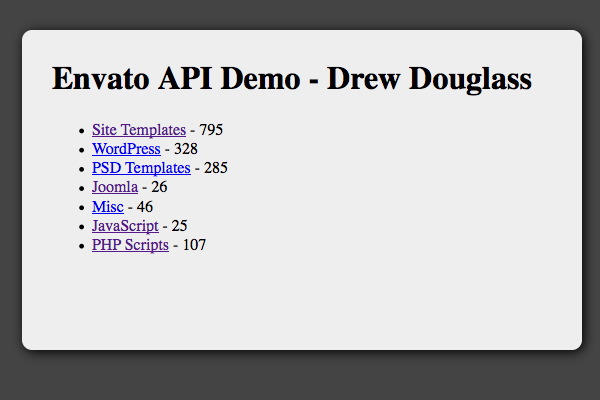
4. New Files
Just like the ThemeForest homepage displays a list of new files, you, too, can access new files from a given marketplace from the using new-files set. A nice addition from this set, is the ability to also display the item thumbnail, as you’ll shortly see.
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://marketplace.envato.com/api/v1/new-files:themeforest,wordpress.json'); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $ch_data = curl_exec($ch); curl_close($ch); if(!empty($ch_data)) { $json_data = json_decode($ch_data, true); //print_r($json_data); $data_count = count($json_data['new-files']) -1; echo '
- ';
for($i = 0; $i <= $data_count; $i++)
{
echo '
',$json_data['new-files'][$i]['item'],'
';
}
echo '
Unlike our previous snippets, this set requires two parameters. It requires the marketplace and the desired category respectively. Notice that we have added some PHP and markup inside of our for loop. This allows us to display a thumbnail of the item.
A sample output of this snippet is below:
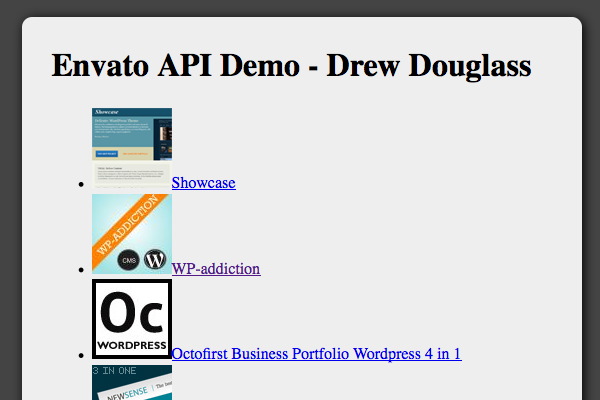
5. Popular
Similar to displaying recently uploaded items to a given marketplace, we can also display popular items from a given marketplace. Perhaps you want to display a list of popular ThemeForest files on your blog. This snippet would accomplish that goal.
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://marketplace.envato.com/api/v1/popular:themeforest.json'); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $ch_data = curl_exec($ch); curl_close($ch); if(!empty($ch_data)) { $json_data = json_decode($ch_data, true); //print_r($json_data); $json_short = $json_data['popular']['items_last_week'];//Save us some typing. $data_count = count($json_short) -1; echo '
- ';
for($i = 0; $i <= $data_count; $i++)
{
echo '
',$json_short[$i]['item'],'
';
}
echo '
Note how we have added an additional variable named json_short to save us some typing while traversing through the nested array of returned data. In just a few lines of code, we are able to display the thumbnail, name, and link of the item as demonstrated below:
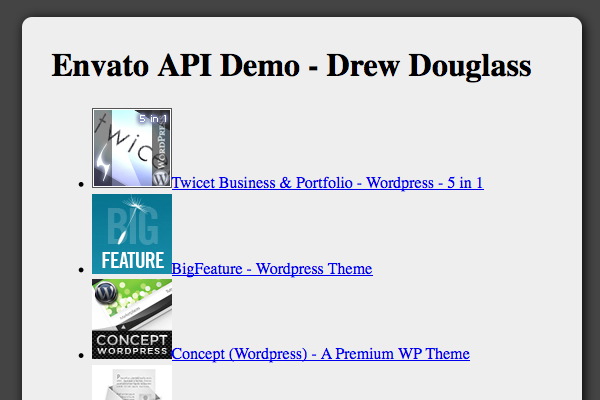
6. New Files From User
The new-files-from-user is a popular set that we used a while back when we created a WordPress plugin with the Envato API. This set allows you to retrieve the 10 newest files a user has uploaded, and the data that goes along with it. You could use this set to promote yourself on your blog automatically every time you upload a new item.
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://marketplace.envato.com/api/v1/new-files-from-user:creatingdrew,themeforest.json'); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $ch_data = curl_exec($ch); curl_close($ch); if(!empty($ch_data)) { $json_data = json_decode($ch_data, true); //print_r($json_data); $data_count = count($json_data['new-files-from-user']) -1; echo '
- ';
for($i = 0; $i <= $data_count; $i++)
{
echo '
',$json_data['new-files-from-user'][$i]['item'],'
';
}
echo '
The parameters required are the username and the marketplace desired. The rest of the snippet follows the same logic that we have been discussing. Below is an example of the output two of my recent files.
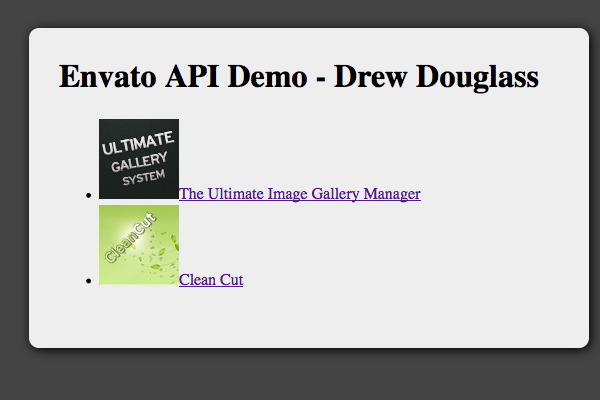
7. Random New Files
The random-new-files set is pretty self explanatory and acts as expected. It returns a random list of newly uploaded files from a given marketplace. It also returns meta data about the file you are free to use and manipulate.
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://marketplace.envato.com/api/v1/random-new-files:themeforest.json'); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $ch_data = curl_exec($ch); curl_close($ch); if(!empty($ch_data)) { $json_data = json_decode($ch_data, true); //print_r($json_data); $data_count = count($json_data['random-new-files']) -1; echo '
- ';
for($i = 0; $i <= $data_count; $i++)
{
echo '
',$json_data['random-new-files'][$i]['item'],'
';
}
echo '
There’s nothing you haven’t seen already here. We are using this set to display some thumbnails and the title. Be sure to check out all the data that is returned though, you may be interested in it. A screenshot of the output is below:
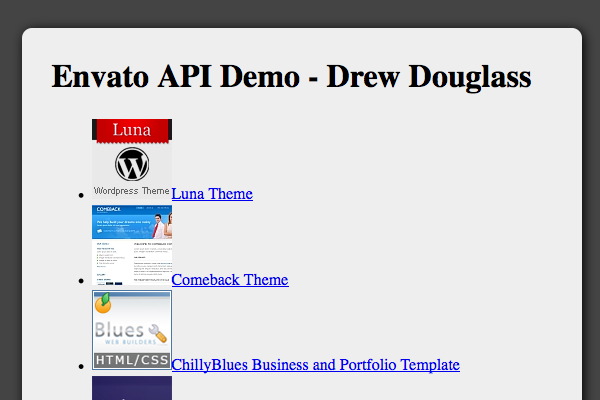
8. Search
That’s right, you can actually use the API to search for custom data in custom categories from custom marketplaces! The devs really thought the API through, and the search set is perfect proof of that. Let’s take a look at a short search snippet. Keep in mind you would could take all this data from user input and setup a custom Envato search on your site, but that is beyond the scope of this tutorial.
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://marketplace.envato.com/api/v1/search:themeforest,wordpress,clean.json'); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $ch_data = curl_exec($ch); curl_close($ch); if(!empty($ch_data)) { $json_data = json_decode($ch_data, true); //print_r($json_data); $data_count = count($json_data['search']) -1; echo '
- ';
for($i = 0; $i <= $data_count; $i++)
{
echo '
- ',$json_data['search'][$i]['description'],' '; } echo '
Above bears some explaining.
- We have changed our request URL to the search set.
- The search set takes three parameters, the marketplace, the category to search, and the term to search for.
- Here, we have searched for the term clean
I recommend checking out the API documentation and check out everything that is possible with the search set.
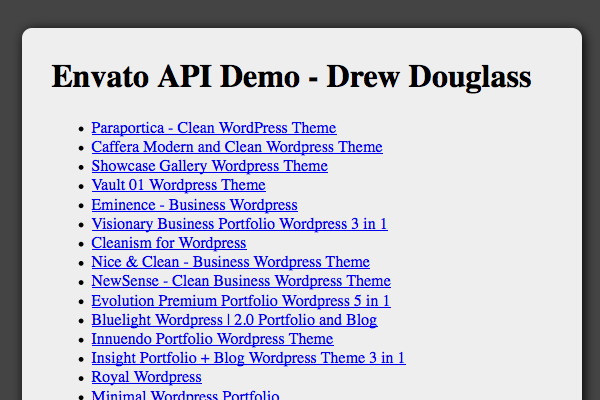
9. User
The user data set returns a small amount of information about a given user. Note that the API key is not required.
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://marketplace.envato.com/api/v1/user:collis.json'); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $ch_data = curl_exec($ch); curl_close($ch); if(!empty($ch_data)) { $json_data = json_decode($ch_data, true); //print_r($json_data); echo '
- ';
echo '
- Location =',$json_data['user']['location'],' '; echo '
- Username =',$json_data['user']['username'],' '; echo '
- Sales =',$json_data['user']['sales'],' '; echo '
Since we are returning information from one user only, there is no need to perform any looping — just output the data.
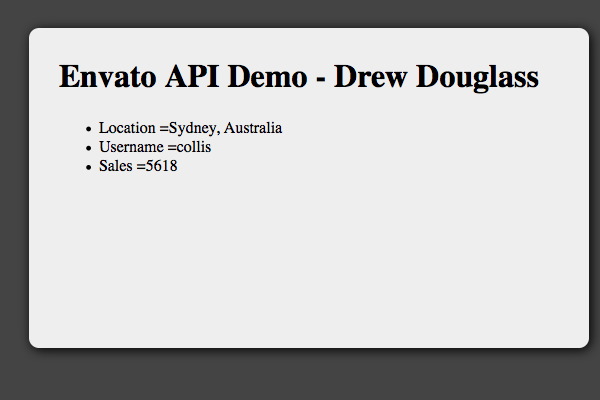
10. Releases
You’ll probably never need to use the releases set, but I said we would cover every public set, and that’s what well do. The releases set returns release and set information for the API. Basically, it is simply used to generate documentation. Just in case you desire the snippet to display this information, you may find it below:
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://marketplace.envato.com/api/v1/releases.json'); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $ch_data = curl_exec($ch); curl_close($ch); if(!empty($ch_data)) { $json_data = json_decode($ch_data, true); print_r($json_data); } else { echo 'Sorry, but there was a problem connecting to the API.'; }
Thanks for Reading
We have covered every single set listed in the public set of v1 of the API! Feel free to pat yourself on the back and go eat some bacon, you deserve it.
This article was originally posted on the ThemeForest blog late last year. We are currently porting over some of the more popular articles to Nettuts+.