In this Quick Tip, you’ll learn how to detect when the user has been inactive for a determined time. Keep reading to find out how!
Final Result Preview
Let’s take a look at the final result we will be working towards:
Step 1: Brief Overview
We’ll detect users interaction using Keyboard and Mouse Events, checking for recent activity using a Timer. We’ll display a message if the determined time has elapsed without activity.
Step 2: Set Up Your Flash File
Launch Flash and create a new Flash Document.
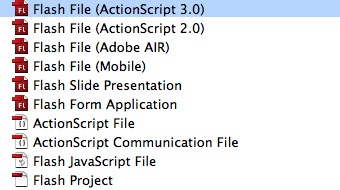
Set the stage size to 550×250px.
Step 3: Interface
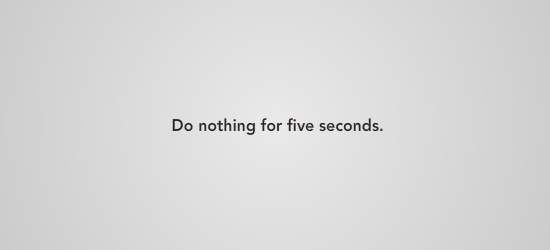
This is the interface we’ll be using, a simple background with a message telling the user to wait for five seconds. The following screen will be shown when the five seconds have passed – convert it to MovieClip and mark the Export for ActionScript box. It’s named TheScreen.
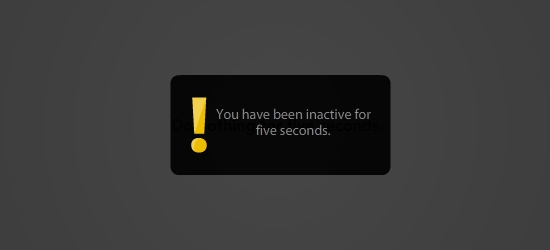
Of course this is only one of the many things you can do when the inactivity time passes, GrooveShark for example, pauses the music and shows a message asking the user if he wants to continue using the application. It’s your call to decide what to do.
Step 4: ActionScript
This is the class that does the work, read the comments in the code to find out about its behavior.
package { import flash.display.Sprite; import flash.events.TimerEvent; import flash.utils.Timer; import flash.events.MouseEvent; import flash.events.KeyboardEvent; public class Main extends Sprite { private var screen:TheScreen = new TheScreen(); //Creates a new instance of TheScreen private var added:Boolean = false; //A boolean to check if the screen has been added to stage /* Timer Object */ private var timer:Timer = new Timer(5000);//Five seconds for this example public function Main():void { timer.start(); //Starts the timer timer.addEventListener(TimerEvent.TIMER, showMsg); //Listens for the timer to complete /* Mouse and keyboard listeners, stops the timer when a event occurs, if you are using other input method, like the microphone, add its event here */ stage.addEventListener(MouseEvent.MOUSE_MOVE, stopTimer); stage.addEventListener(MouseEvent.MOUSE_DOWN, stopTimer); stage.addEventListener(MouseEvent.MOUSE_UP, stopTimer); stage.addEventListener(KeyboardEvent.KEY_DOWN, stopTimerK); stage.addEventListener(KeyboardEvent.KEY_UP, stopTimerK); } /* If there is no activity for 5 seconds, a message will display */ private function showMsg(e:TimerEvent):void { addChild(screen); //Adds the screen added = true; } /* If there's activity, we clear the message and reset the timer */ private function stopTimer(e:MouseEvent):void { if (added) { removeChild(screen); added = false; } timer.stop(); timer.start(); } private function stopTimerK(e:KeyboardEvent):void { if (added) { removeChild(screen); added = false; } timer.stop(); timer.start(); } } }
Step 5: Document Class
Remember to add the class name to the Class field in the Publish section of the Properties panel.
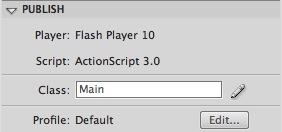
Conclusion
This is a useful and easy way to detect user activity. In this example the activity detected is based in the Mouse and Keyboard events, but you can easily add a Microphone or other input event to meet your needs.
I hope you liked this Quick Tip, thanks for reading!